I have been making some modifications to my forms to prepare for New Teams limitations and have noticed that the data table delete button generates an alert making it impossible to delete data table items while working on a form within teams. I have managed to get rid of the confirmation using:
fd.control('DataTable').widget.setOptions({
editable: {
confirmation: false
}
});
I was wondering if it would be possible to create a modal dialog using an HTML element to confirm the row deletion? I am not sure how to go about deleting the row.
Hello @cwalter2,
Developers think that it can be fixed with the code. It's a bit tricky so they need some time to prepare it.
I will write to you once they share the code with me.
@cwalter2,
Here is code that can be used to display a prompt in new Teams when a user deletes a row in a data table:
const dataTableId = 'DataTable1';
function bindHandlers() {
fd.control(dataTableId)
.widget.wrapper
.find("tbody>tr:not(.k-detail-row,.k-grouping-row):visible a.k-grid-delete")
.off('click')
.on('click', customDelete);
}
function customDelete(e) {
e.stopPropagation();
e.preventDefault();
const deleteConfirmationDialog = $("#confirmation-window").kendoDialog({
title: 'Confirmation',
content: "Are you sure you want to delete the row?",
visible: false,
actions: [
{ text: 'Cancel' },
{
text: 'Delete',
primary: true,
action: () => {
const row = $(e.currentTarget).parent().parent();
fd.control(dataTableId).widget.removeRow(row);
}
}
]
});
const dialog = deleteConfirmationDialog.data("kendoDialog");
dialog.open();
return false;
}
fd.rendered(() => {
fd.control(dataTableId).widget.setOptions({
editable: { confirmation: false }
});
bindHandlers();
fd.control(dataTableId).widget.bind("dataBound", bindHandlers);
});
And you need to add a HTML code like so to the form:
<div id= "confirmation-window"> </div>
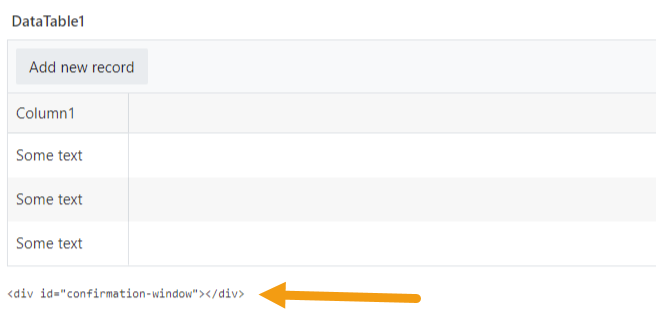
That works perfectly. Is there a way to reuse the code for multiple datatables on the same form? I have tried a couple of ideas and have come up short.
@cwalter2,
You can use this code for multiple Data Tables:
const dataTableIds = ['DataTable1', 'DataTable2', 'DataTable3'];
const openConfirmationDialog = (e, dataTableId) => {
const deleteConfirmationDialog = $("#confirmation-window").kendoDialog({
title: 'Confirmation',
content: "Are you sure you want to delete the row?",
visible: false,
actions: [{
text: 'Cancel'
},
{
text: 'Delete',
primary: true,
action: () => {
const row = $(e.currentTarget).parent().parent();
fd.control(dataTableId).widget.removeRow(row);
}
}
]
});
const dialog = deleteConfirmationDialog.data("kendoDialog");
dialog.open();
}
fd.rendered(() => {
dataTableIds.forEach(dataTableId => {
fd.control(dataTableId).widget.setOptions({
editable: {
confirmation: false
}
});
const customDelete = (e) => {
e.stopPropagation();
e.preventDefault();
openConfirmationDialog(e, dataTableId);
return false;
}
const bindHandlers = () => {
fd.control(dataTableId)
.widget.wrapper
.find("tbody>tr:not(.k-detail-row,.k-grouping-row):visible a.k-grid-delete")
.off('click')
.on('click', customDelete);
}
bindHandlers();
fd.control(dataTableId).widget.bind("dataBound", bindHandlers);
})
});
1 Like