Hello,
I know this has been posted several times but I’m having trouble using multiple lists in my environment. I’ve followed your blog post about using multiple lists and have created a “Project List” (attached). I have gotten to step 5 (5.Click ‘Process’) and I don’t see the categories from the other lists as noted in Step 6.
The one difference with your example is that I’m using a SharePoint List versus a Task List. I have named the Project List list the same. I also have a “Status” column in the project lists. Can you help guide me in the right direction?
[code]//…
handlers.requestSuccess = function(data, logger) {
var dfd = $.Deferred();
var projectList = data.items;
data.items = [];
var deferreds = [];
var query = new SP.CamlQuery(’
10000 ');
for (var project in projectList){
var d = request(query, projectList[project],
‘SPList’, [‘ID’, ‘Status’], data);
deferreds.push(d);
}
console.log(‘All requests fired.’);
$.when.apply(null, deferreds).done(function() {
console.log(‘All requests have been resolved.’);
dfd.resolve();
});
return dfd.promise();
}
//…
function request(query, project, listName, fields, data) {
var dfd = $.Deferred();
var ctx = new SP.ClientContext(project['URL']);
var list = ctx.get_web().get_lists().getByTitle(listName);
var itemCollection = list.getItems(query);
ctx.load(itemCollection, 'Include(' + fields.join(',') + ')');
ctx.executeQueryAsync(function () {
var itemCollectionEnumerator = itemCollection.getEnumerator();
while (itemCollectionEnumerator.moveNext()) {
var listItem = itemCollectionEnumerator.get_current();
var item = {};
$.each(fields, function () {
try {
var value = listItem.get_item(this);
item[this] = value;
}
catch (e) { }
});
item['Project'] = project['Title'];
data.items.push(item);
}
console.log('Request to ' + project['URL'] + ' has been resolved.');
dfd.resolve();
}, function(caller, args){
console.log('Error: ' + args.get_message());
});
return dfd.promise();
}
[/code]
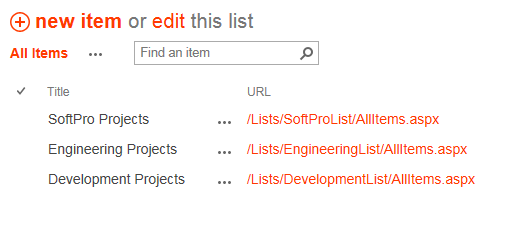
Hi,
You need to aggregate data from multiple lists from the same site and you store list URLs (not site URLs as in our sample) in the Project List, am I right?
Yes, you are correct. I have used the list URLs and all lists are on the same site.
So, what you’re doing is slightly different to what the blog post describes, so you’ll need to make the following changes (at least, as I haven’t tested this):
- In the Project list you need to put the site URL of the site, relative to the site collection. If it’s a root site, then just put /, it should work
- Add another column to the Projects list, call it “ListName”. Add respective names of corresponding lists here.
-
change the signature of request from:
function request(query, project, listName, fields, data) { ... }
to
function request(query, project, fields, data) { ... }
change:
var list = ctx.get_web().get_lists().getByTitle(listName);
to:
var list = ctx.get_web().get_lists().getByTitle(project['ListName']);
change:
var d = request(query, projectList[project], 'Task List', ['ID', 'Status'], data);
to:
var d = request(query, projectList[project], ['ID', 'Status'], data);
I have made the changes as I understand what you’re asking.
The ProjectList list contains two columns and three rows because I have three lists
- Titled ListName that contains the name of the list
- the URL of the site housing the list (the image shows the description of the URL but the actual URL is there)
I have made your code changes and again it does not work. I am actually not understanding why my task is different from the example you have in the blog post.
Any thoughts on what else I can do?
Code:[code]//…
handlers.requestSuccess = function(data, logger) {
var dfd = $.Deferred();
var projectList = data.items;
data.items = [];
var deferreds = [];
var query = new SP.CamlQuery(’
10000 ');
for (var project in projectList){
var d = request(query, projectList[project], [‘ID’, ‘Status’], data);
deferreds.push(d);
}
console.log(‘All requests fired.’);
$.when.apply(null, deferreds).done(function() {
console.log(‘All requests have been resolved.’);
dfd.resolve();
});
return dfd.promise();
}
//…
function request(query, project, fields, data) {…}
var dfd = $.Deferred();
var ctx = new SP.ClientContext(project['URL']);
var list = ctx.get_web().get_lists().getByTitle(project['ListName']);
var itemCollection = list.getItems(query);
ctx.load(itemCollection, 'Include(' + fields.join(',') + ')');
ctx.executeQueryAsync(function () {
var itemCollectionEnumerator = itemCollection.getEnumerator();
while (itemCollectionEnumerator.moveNext()) {
var listItem = itemCollectionEnumerator.get_current();
var item = {};
$.each(fields, function () {
try {
var value = listItem.get_item(this);
item[this] = value;
}
catch (e) { }
});
item['Project'] = project['Title'];
data.items.push(item);
}
console.log('Request to ' + project['URL'] + ' has been resolved.');
dfd.resolve();
}, function(caller, args){
console.log('Error: ' + args.get_message());
});
return dfd.promise();
}
[/code]
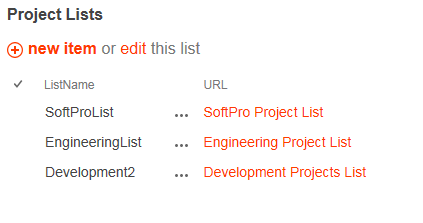
- Try to switch the type of the URL column to Single line of text.
- If you store all the lists at the same site (the same site as where the Project Lists is located), please, try the code below:
[code]//…
handlers.requestSuccess = function(data, logger) {
var dfd = $.Deferred();
var projectList = data.items;
data.items = [];
var deferreds = [];
var query = new SP.CamlQuery(’
10000 ');
for (var project in projectList){
var d = request(query, projectList[project], [‘ID’, ‘Status’], data);
deferreds.push(d);
}
console.log(‘All requests fired.’);
$.when.apply(null, deferreds).done(function() {
console.log(‘All requests have been resolved.’);
dfd.resolve();
});
return dfd.promise();
}
//…
function request(query, project, fields, data) {…}
var dfd = $.Deferred();
var ctx = SP.ClientContext.get_current();
var list = ctx.get_web().get_lists().getByTitle(project['ListName']);
var itemCollection = list.getItems(query);
ctx.load(itemCollection, 'Include(' + fields.join(',') + ')');
ctx.executeQueryAsync(function () {
var itemCollectionEnumerator = itemCollection.getEnumerator();
while (itemCollectionEnumerator.moveNext()) {
var listItem = itemCollectionEnumerator.get_current();
var item = {};
$.each(fields, function () {
try {
var value = listItem.get_item(this);
item[this] = value;
}
catch (e) { }
});
item['Project'] = project['Title'];
data.items.push(item);
}
console.log('Request to ' + project['ListName'] + ' has been resolved.');
dfd.resolve();
}, function(caller, args){
console.log('Error: ' + args.get_message());
});
return dfd.promise();
}[/code]
- If both do not help, please, pay for the support and provide Project List template (List settings -> Save list as template) and the chart’s configuration (Export it in Dashboard Designer):
plumsail.com/sharepoint-dashboa … y=11274284
As I can see, you have purchased the support minutes. Please, send the list template and the configuration of the chart to support@spchart.com.